Python Math Fundamentals
How strong is your Python Math foundation? Do a quick review of the basics. Then download and try out the 1-page Python Math Quiz!
How strong is your Python Math foundation? Do a quick review of the basics. Then download and try out the 1-page Python Math Quiz!
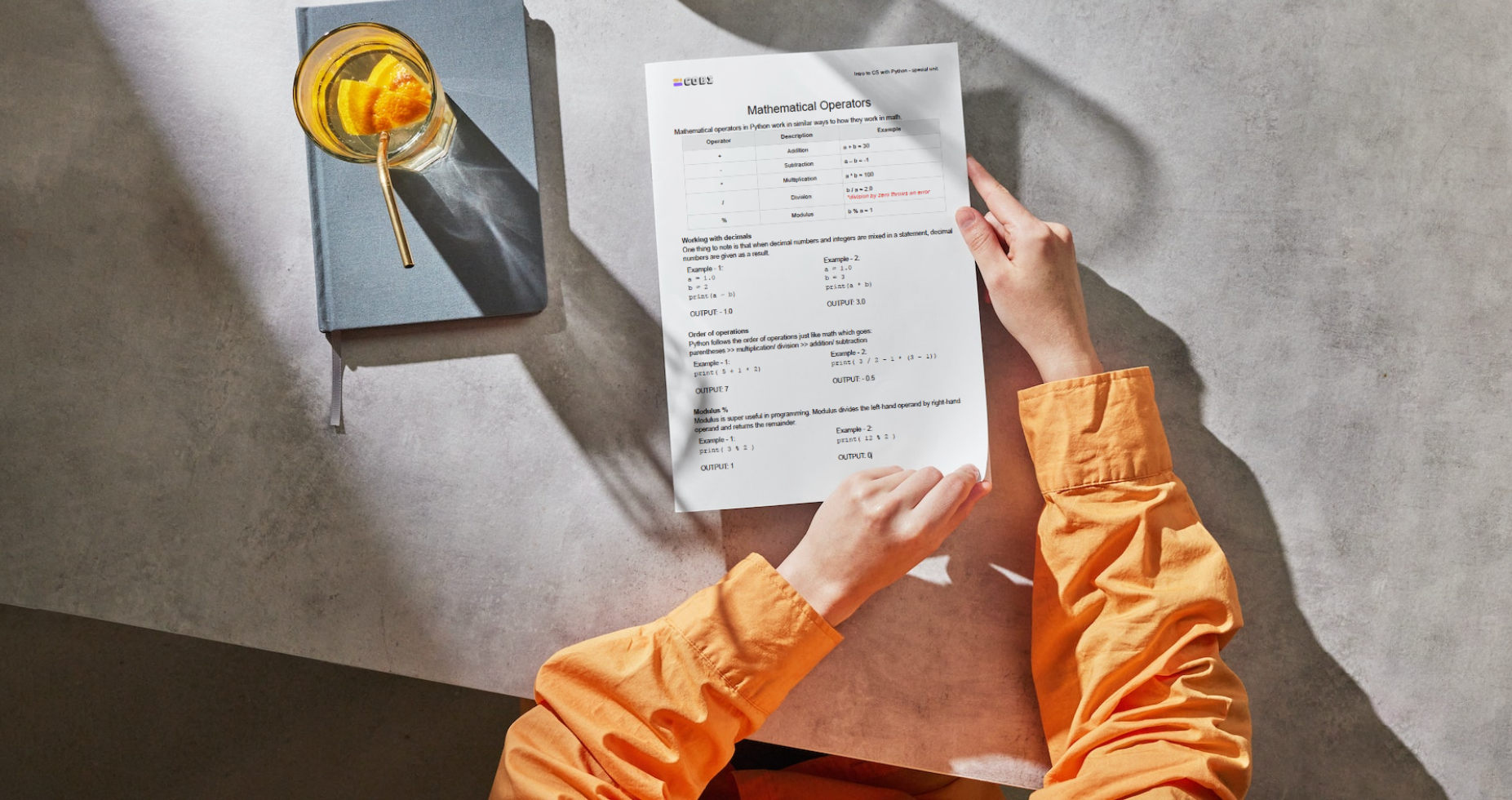
Mathematical Operators
Mathematical operators in Python work in similar ways to how they work in math.
Working with decimals
One thing to note is that when decimal numbers and integers are mixed in a statement, decimal numbers are given as a result.
Order of operations
Python follows the order of operations just like math which goes:
parentheses >> multiplication/ division >> addition/ subtraction
Modulus %
Modulus is super useful in programming. Modulus divides the left-hand operand by the right-hand operand and returns the remainder.
Related Links: