Scratch to Java (Part-1)
Transitioning from block coding to text can be challenging. In this post, we'll look into the 1:1 code comparison of Scratch and Java. In the next post, we'll take a Scratch project and code it in Java.
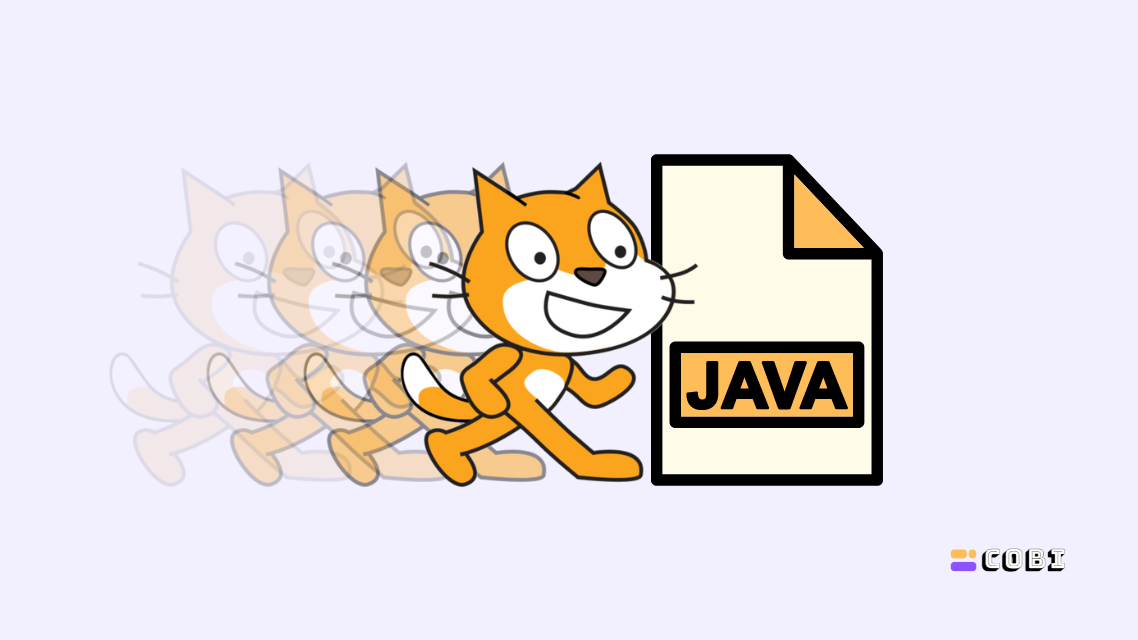
Transitioning from block coding to text can be challenging. In this post, we'll look into the 1:1 code comparison of Scratch and Java. In the next post, we'll take a Scratch project and code it in Java.
Common challenges of transitioning from Scratch to Java:
- All code blocks are visible on Scratch. However, for Java, students will have to look up the Java syntax and commands from documentation websites or by searching online.
- Scratch doesn't produce syntax errors. Java on the other hand has strict syntax rules and the wrong syntax will result in errors.
- Scratch produces visual results and animations. Students may find Java less engaging at first.
Level of Abstraction
Block-based program | ![]() |
High-level program (Java) | class Triangle { … double area(float b, float h) { return b*h/2; } ... } |
Low-level program | LOAD r1, b LOAD r2, h MUL r1, r2 DIV r1, #2 RET |
Executable Machine code | 0000100101010001010001010 1000101010000110101001100 0101001000100010010101000 1010001010100010101000... |
Scratch to Java Code Examples
Output
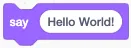
System.out.println("Hello World!");
Instantiating a variable
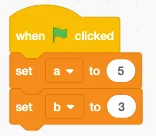
int a = 5;
int b = 3;
Operators
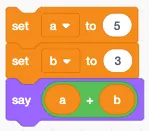
int a = 5;
int b = 3;
System.out.println(a + b);
Conditional Statements
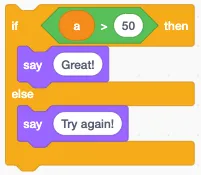
if (a > 50) {
System.out.println("Great!");
}else{
System.out.println("Try again!");
}
For loop
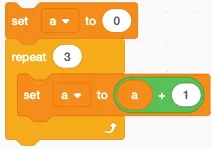
int a = 0;
for (int i = 0; i < 3; i++){
a = a + 1;
}
Getting user input
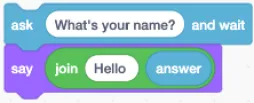
import java.util.Scanner;
public class Test {
public static void main(String[] args){
Scanner scanObj = new Scanner(System.in);
System.out.println("What's your name?");
String name = scanObj.nextLine();
System.out.println("Hi "+ name);
}
}
Function and function call
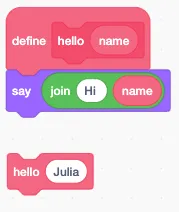
public static void main(String[] args){
hello("Julia");
}
public static void hello(String name){
System.out.println("Hello " + name);
}
Related Links: